Many of you would have faced this error while executing a SQL Query. Below are the details on this error.
ORA-00936:
missing expression
Cause:
A required part of a clause or expression has been omitted. For example, a SELECT statement may have been entered without a list of columns or expressions or with an incomplete expression. This message is also issued in cases where a reserved word is misused, as in SELECT TABLE.
The common reasons for this error are:
- You tried to assign a value to a numeric variable, but the value is larger than the variable can handle.
- You tried to assign a non-numeric value to a numeric variable and caused a conversion error.
Action:
Check the statement syntax and specify the missing component.
Example :
SELECT STUDENT_ID,STUDENT_NAME, FROM STUDENTS_TABLE;
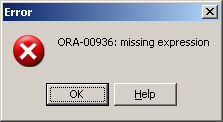
if you notice in the above SELECT statement there is extra comma after STUDENT_NAME due to which this error occured.